falling cat
y <- rep(NA, 20) # empty vector to store result
i <- 1 # time
y[i] <- 10 # initial vertical position
while(y[i]>0) { # while we don't hit the floor...
i <- i+1 # time passes
y[i] <- y[i-1] + -1 # update position
} # ..and repeat
plot(y) # draw all positions
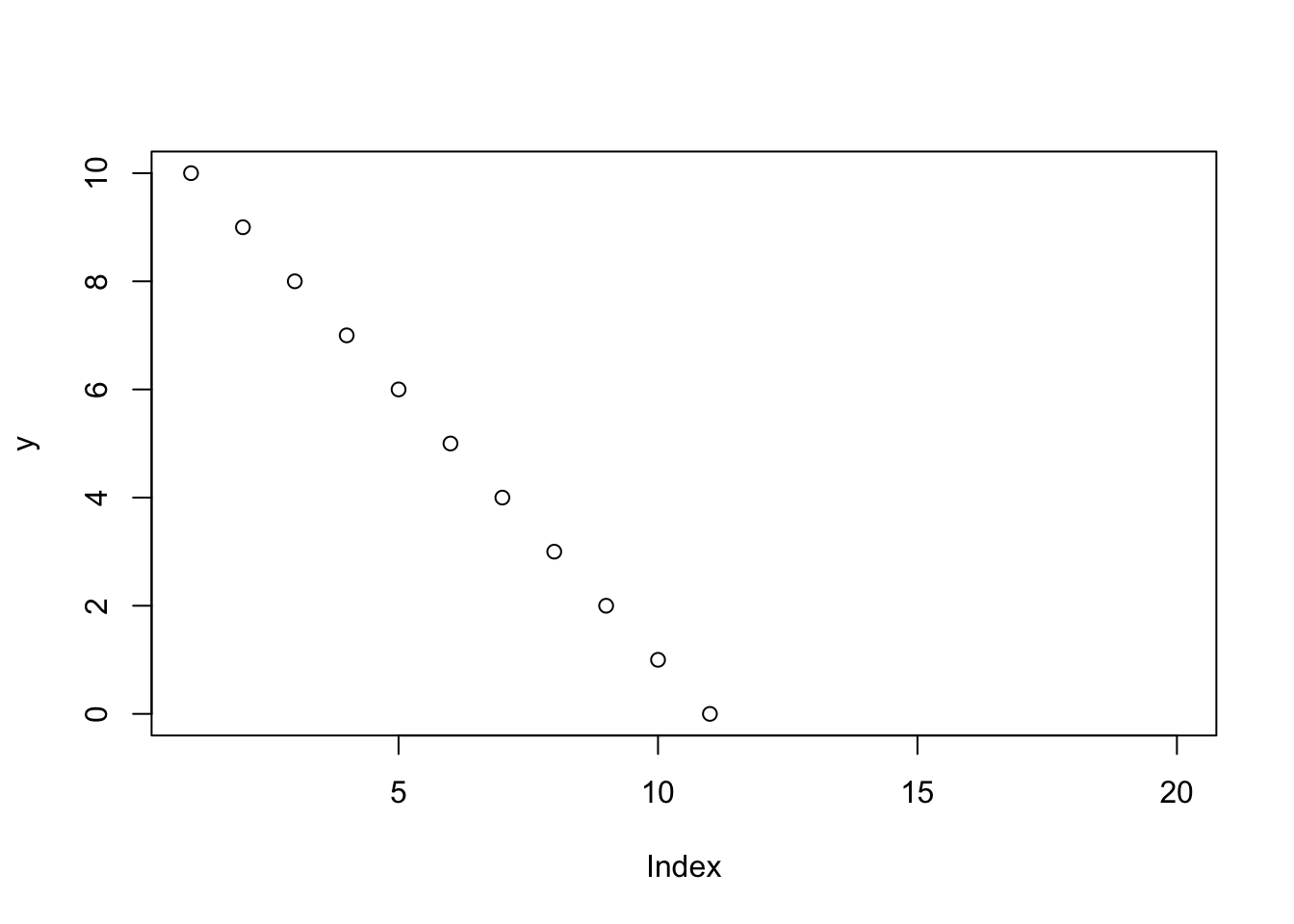
Bounce
N <- 60
y <- rep(NA, N) # initialize to empty
y[1] <- 120 # initial position
speed <- -20 # initial speed
for(i in 2:N) { # as time passes...
y[i] <- y[i-1] + speed # update position
if(y[i] < -180){ # if we hit the floor
speed <- -1*speed # bounce
}
if(y[i] > 180){ # if we hit the ceil
speed <- -1*speed # bounce
}
}
plot(y)
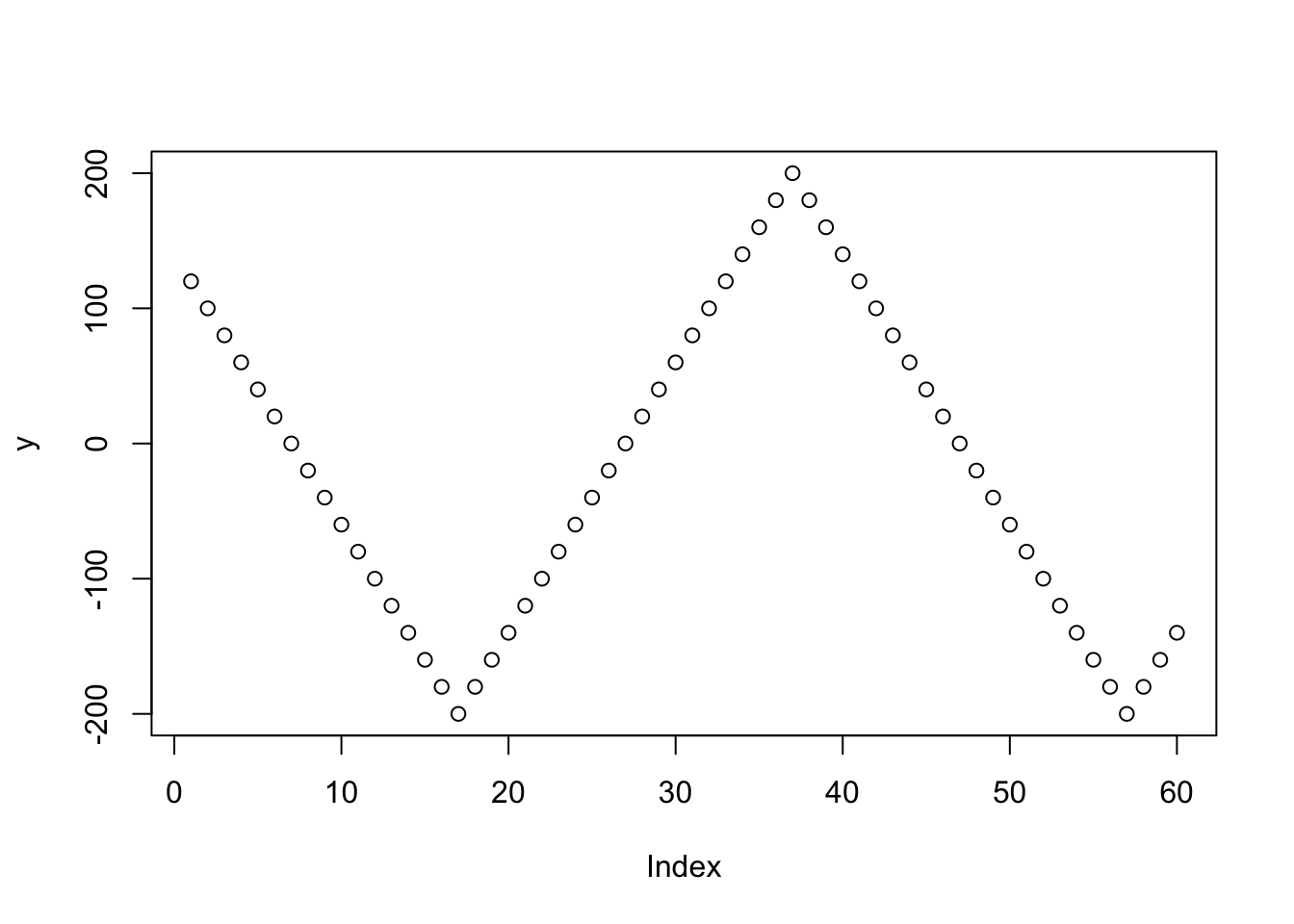
Boolean bounce
N <- 60
y <- rep(NA, N) # initialize to empty
y[1] <- 120 # initial position
speed <- -20 # initial speed
for(i in 2:N) { # as time passes...
y[i] <- y[i-1] + speed # update position
if(y[i] < -180 | y[i] > 180){ # if we hit the floor or ceil
speed <- -1*speed # bounce
}
}
plot(y)
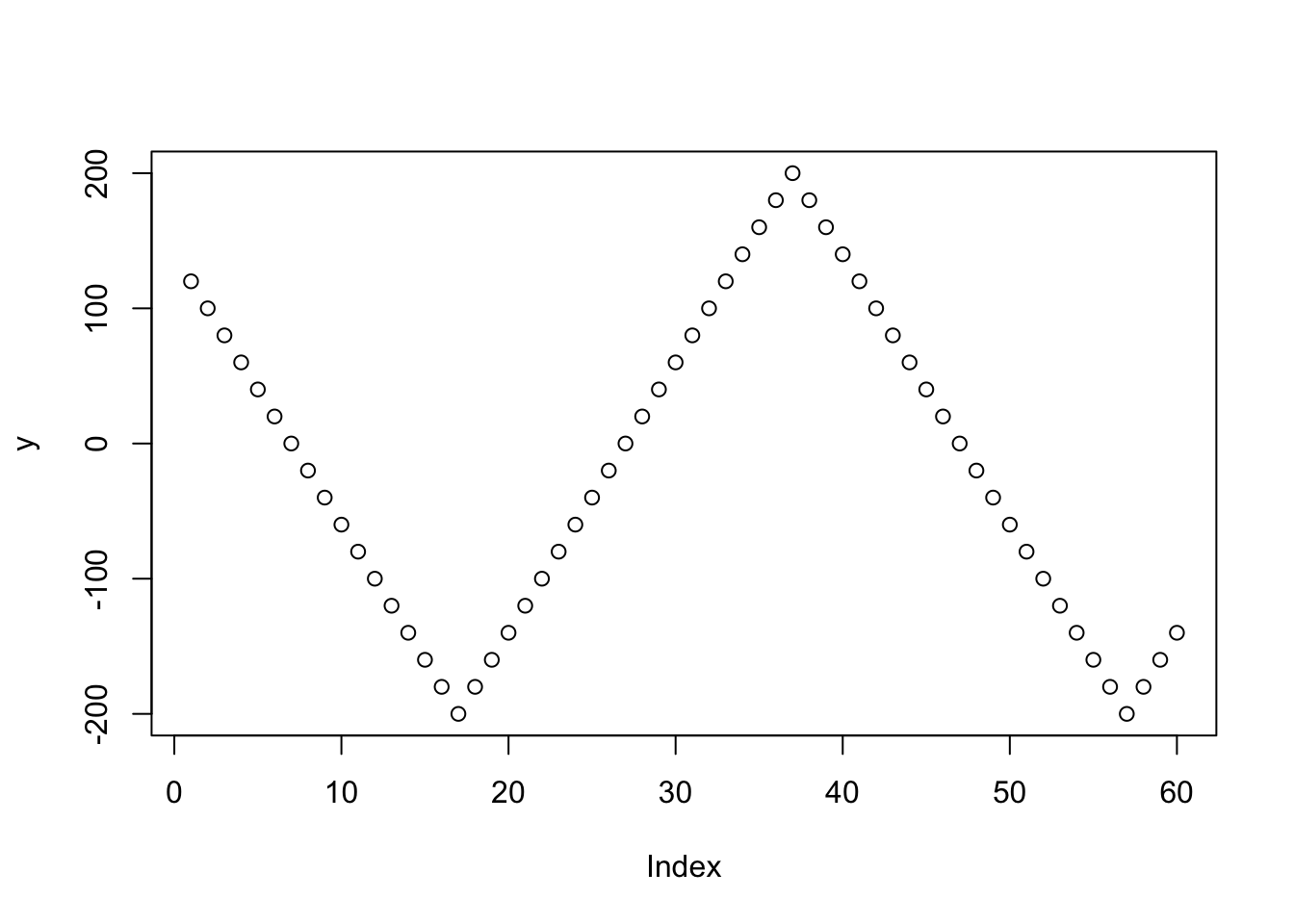
gravity
N <- 60
y <- rep(NA, N) # initialize to empty
y[1] <- 120 # initial position
speed <- -20 # initial speed
for(i in 2:N) { # as time passes...
y[i] <- y[i-1] + speed # update position
if(y[i] < -180 | y[i] > 180){ # if we hit the floor or ceil
speed <- -1*speed # bounce
}
}
plot(y)
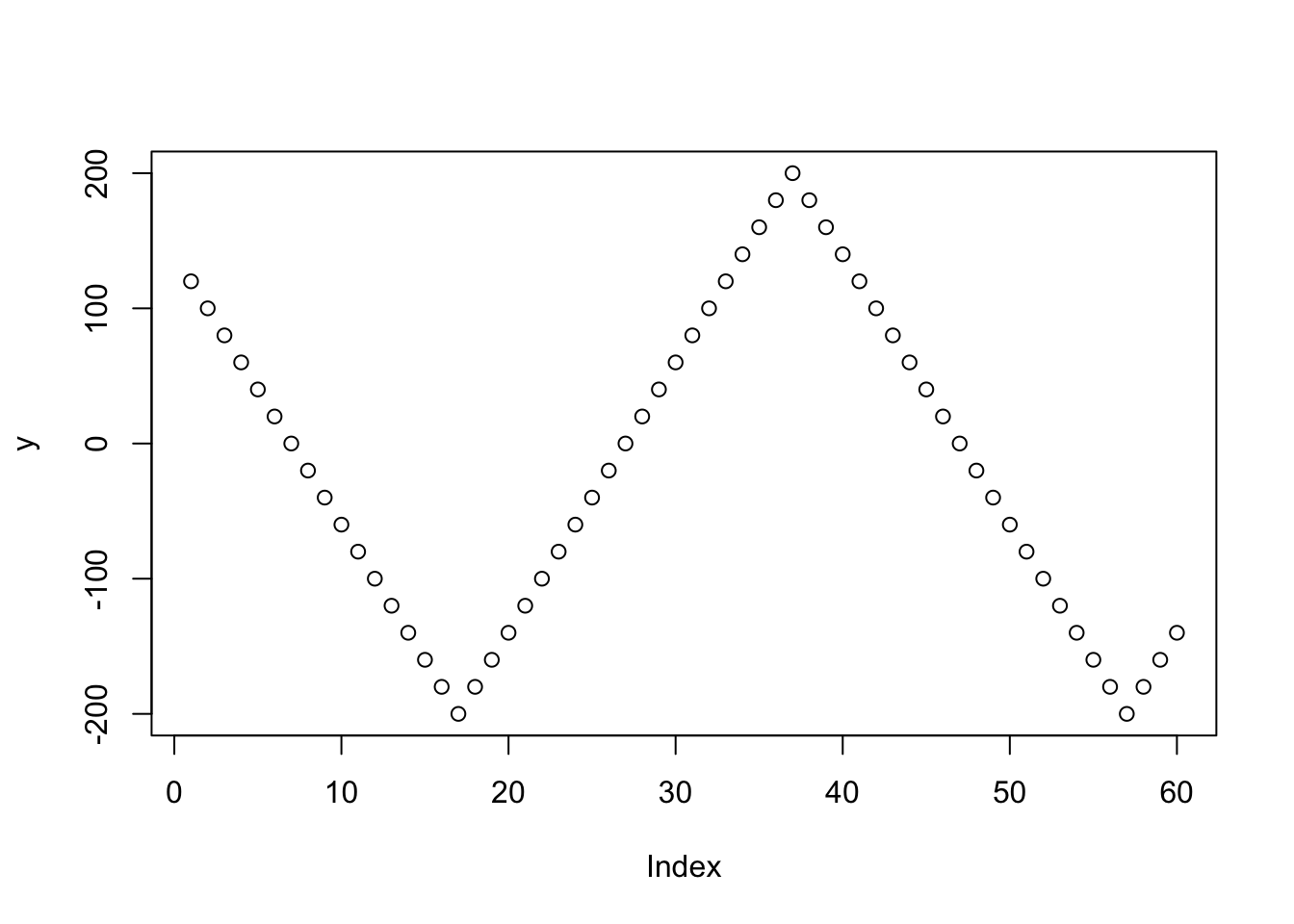